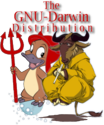
|
Some people complain that Allegro produces very large executables. This is
certainly true: with the djgpp version, a simple "hello world" program will
be about 200k, although the per-executable overhead is much less for
platforms that support dynamic linking. But don't worry, Allegro takes up a
relatively fixed amount of space, and won't increase as your program gets
larger. As George Foot so succinctly put it, anyone who is concerned about
the ratio between library and program code should just get to work and write
more program code to catch up :-)
Having said that, there are several things you can do to make your programs
smaller:
-
For all platforms, you can use an executable compressor called UPX, which
is available at http://upx.tsx.org/ . This usually manages a compression
ratio of about 40%.
-
When using djgpp: for starters, read the djgpp FAQ section 8.14, and take
note of the -s switch. And don't forget to compile your program with
optimisation enabled!
-
If a DOS program is only going to run in a limited number of graphics modes,
you can specify which graphics drivers you would like to include with the
code:
BEGIN_GFX_DRIVER_LIST
driver1
driver2
etc...
END_GFX_DRIVER_LIST
where the driver names are any of the defines:
GFX_DRIVER_VBEAF
GFX_DRIVER_VGA
GFX_DRIVER_MODEX
GFX_DRIVER_VESA3
GFX_DRIVER_VESA2L
GFX_DRIVER_VESA2B
GFX_DRIVER_XTENDED
GFX_DRIVER_VESA1
This construct must be included in only one of your C source files. The
ordering of the names is important, because the autodetection routine works
down from the top of the list until it finds the first driver that is able
to support the requested mode. I suggest you stick to the default ordering
given above, and simply delete whatever entries you aren't going to use.
-
If your DOS program doesn't need to use all the possible color depths, you
can specify which pixel formats you want to support with the code:
BEGIN_COLOR_DEPTH_LIST
depth1
depth2
etc...
END_COLOR_DEPTH_LIST
where the color depth names are any of the defines:
COLOR_DEPTH_8
COLOR_DEPTH_15
COLOR_DEPTH_16
COLOR_DEPTH_24
COLOR_DEPTH_32
Removing any of the color depths will save quite a bit of space, with the
exception of the 15 and 16 bit modes: these share a great deal of code, so
if you are including one of them, there is no reason not to use both. Be
warned that if you try to use a color depth which isn't in this list, your
program will crash horribly!
-
In the same way as the above, you can specify which DOS sound drivers you
want to support with the code:
BEGIN_DIGI_DRIVER_LIST
driver1
driver2
etc...
END_DIGI_DRIVER_LIST
using the digital sound driver defines:
DIGI_DRIVER_SOUNDSCAPE
DIGI_DRIVER_AUDIODRIVE
DIGI_DRIVER_WINSOUNDSYS
DIGI_DRIVER_SB
and for the MIDI music:
BEGIN_MIDI_DRIVER_LIST
driver1
driver2
etc...
END_MIDI_DRIVER_LIST
using the MIDI driver defines:
MIDI_DRIVER_AWE32
MIDI_DRIVER_DIGMID
MIDI_DRIVER_ADLIB
MIDI_DRIVER_MPU
MIDI_DRIVER_SB_OUT
If you are going to use either of these sound driver constructs, you must
include both.
-
Likewise for the DOS joystick drivers, you can declare an inclusion list:
BEGIN_JOYSTICK_DRIVER_LIST
driver1
driver2
etc...
END_JOYSTICK_DRIVER_LIST
using the joystick driver defines:
JOYSTICK_DRIVER_WINGWARRIOR
JOYSTICK_DRIVER_SIDEWINDER
JOYSTICK_DRIVER_GAMEPAD_PRO
JOYSTICK_DRIVER_GRIP
JOYSTICK_DRIVER_STANDARD
JOYSTICK_DRIVER_SNESPAD
JOYSTICK_DRIVER_PSXPAD
JOYSTICK_DRIVER_N64PAD
JOYSTICK_DRIVER_DB9
JOYSTICK_DRIVER_TURBOGRAFX
JOYSTICK_DRIVER_IFSEGA_ISA
JOYSTICK_DRIVER_IFSEGA_PCI
JOYSTICK_DRIVER_IFSEGA_PCI_FAST
The standard driver includes support for the dual joysticks, increased
numbers of buttons, Flightstick Pro, and Wingman Extreme, because these are
all quite minor variations on the basic code.
-
If you are _really_ serious about this size, thing, have a look at the top
of include/allegro/alconfig.h and you will see the lines:
#define ALLEGRO_COLOR8
#define ALLEGRO_COLOR16
#define ALLEGRO_COLOR24
#define ALLEGRO_COLOR32
If you comment out any of these definitions and then rebuild the library,
you will get a version without any support for the absent color depths,
which will be even smaller than using the DECLARE_COLOR_DEPTH_LIST() macro.
Removing the ALLEGRO_COLOR16 define will get rid of the support for both 15
and 16 bit hicolor modes, since these share a lot of the same code.
Note: the aforementioned methods for removing unused hardware drivers only
apply to statically linked versions of the library, eg. DOS. On Windows and
Unix platforms, you can build Allegro as a DLL or shared library, which
prevents these methods from working, but saves so much space that you
probably won't care about that. Removing unused color depths from alconfig.h
will work on any platform, though.
If you are distributing a copy of the setup program along with your game,
you may be able to get a dramatic size reduction by merging the setup code
into your main program, so that only one copy of the Allegro routines will
need to be linked. See setup.txt for details. In the djgpp version, after
compressing the executable, this will probably save you about 200k compared
to having two separate programs for the setup and the game itself.
|