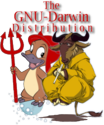
|
SWIG Internals Manual
Thien-Thi Nguyen
ttn@glug.org
David M. Beazley
beazley@cs.uchicago.edu
$Header: /cvs/projects/SWIG/Doc/Devel/Attic/internals.html,v 1.1.2.4 2001/07/30 12:14:44 mkoeppe Exp $
(Note : This is a work in progress.)
Table of Contents
1. Introduction
This document details SWIG internals: architecture and sometimes
implementation. The first few sections concentrate on data structures,
interfaces, conventions and code shared by all language targets.
Subsequent sections focus on a particular language.
The audience is assumed to be SWIG developers (who should also read the
SWIG Engineering Manual before starting
to code).
1.1 Directory Guide
Doc |
HTML documentation. If you find a documentation bug, please
let us know. |
Examples |
This subdir tree contains examples of using SWIG w/ different
scripting languages, including makefiles. Typically, there are the
"simple" and "matrix" examples, w/ some languages offering additional
examples. The GIFPlot example has its own set of per-language
subdirectories. See the README more index.html file in each directory
for more info. [FIXME: Ref SWIG user manual.] |
Lib |
These are the .i (interface) files that form the SWIG
installed library. Language-specific files are in subdirectories (for
example, guile/typemaps.i). Each language also has a .swg file
implementing runtime type support for that language. The SWIG library
is not versioned. |
Misc |
Currently this subdir only contains file fileheader. See
the Engineering Manual for more
info. |
Runtime |
This subdir contains scripts and a makefile for creating runtime
shared-object libraries used by various languages. Runtime/make.sh
says: "The runtime libraries are only needed if you are building
multiple extension modules that need to share information." |
Source |
SWIG source code is in this subdir tree. Directories marked w/ "(*)"
are used in building the swig executable.
DOH (*) |
C library providing memory allocation, file access and generic
containers. Result: libdoh.a |
Experiment |
[TODO] |
Include (*) |
Configuration .h files |
LParse |
Parser (lex / yacc) files and support [why not (*)?!] |
Modules |
[TODO] |
Modules1.1 (*) |
Language-specific callbacks that does actual code generation (each
language has a .cxx and a .h file). Result: libmodules11.a |
Preprocessor (*) |
SWIG-specialized C/C++ preprocessor. Result: libcpp.a |
SWIG1.1 (*) |
Parts of SWIG that are not language-specific, including option
processing and the type-mapping system. Result: libswig11.a.
Note: This directory is currently being phased out. |
SWIG1.3 |
[TODO] [funny, nothing here is presently used for swig-1.3].
This directory might turn into a compatibility interface between
SWIG1.3 and the SWIG1.1 modules. |
Swig (*) |
This directory contains the new ANSI C core of the system
and contains generic functions related to types, file handling,
scanning, and so forth. |
|
Tools |
Libtool support and the mkdist.py script. |
Win |
This improperly-named (spit spit) subdir only has README.txt. |
1.2 Overall Program Flow
Here is the general control flow and where under subdir Source
to look for code:
- Modules1.1/swigmain.cxx:main() is the program entry
point. It parses the language-specifying command-line option (for
example, -java), creating a new language-specific wrapping
object (each language is a C++ class derived from base class
Language). This object and the command-line is passed to
SWIG_main(), whose return value is the program exit value.
- SWIG1.1/main.cxx:SWIG_main() is the "real" main. It
initializes the preprocessor and typemap machinery, defines some
preprocessor symbols, locates the SWIG library, processes common
command-line options, and then calls the language-specific command-line
parser. From here there are three paths: "help", "checkout" and
everything else.
- In "help" mode, clean up open files and exit.
- In "checkout" mode, copy specified files from the SWIG library
to the current directory. Errors cause error messages but no
non-lcoal exits.
- Otherwise, do wrapping: determine output file name(s), define
some preprocessor symbols and run the preprocessor, initialize
the interface-definition parser, set up the typemap for handling
new return strings, and finally do the language-specific parse
(by calling the language object's parse() method), which
creates output files by side-effect.
Afterwards, remove temporary files, and clean up. If the command-line
included -freeze, go into an infinite loop; otherwise return the
error count.
- The language-specific parse() (and all other
language-specific code) lives in Modules1.1/foo.{h,cxx} for
language Foo. Typically, FOO::parse() calls
FOO::headers() and then the global function yyparse(),
which uses the callbacks registered by SWIG_main() above.
2. DOH
DOH is a collection of low-level objects such as strings, lists, and
hash tables upon which the rest of SWIG is built. The name 'DOH'
unofficially stands for "Dave's Object Hack", but it's also a good
expletive to use when things don't work (as in "SWIG core
dumped---DOH!").
2.1 Motivation and Background
The development of DOH is influenced heavily by the problems
encountered during earlier attempts to create a C++ based version of
SWIG2.0. In each of these attempts (over a 3 year period), the
resulting system always ended up growing into a collossal nightmare of
large inheritance hierarchies and dozens of specialized classes for
different types of objects (functions, variables, constants, etc.).
The end result was that the system was tremendously complicated,
difficult to understand, difficult to maintain, and fairly inflexible
in the grand scheme of things.
DOH takes a different approach to tackling the complexity problem.
First, rather than going overboard with dozens of types and class
definitions, DOH only defines a handful of simple yet very useful
objects that are easy to remember. Second, DOH uses dynamic
typing---one of the features that make scripting languages so useful
and which make it possible to accomplish things with much less code.
Finally, DOH utilizes a few coding tricks that allow it to perform
a limited form of function overloading for certain C datatypes (more
on that a little later).
The key point to using DOH is that instead of thinking about code in
terms of highly specialized C data structures, just about everything
ends up being represented in terms of a just a few datatypes. For
example, structures are replaced by DOH hash tables whereas arrays are
replaced by DOH lists. At first, this is probably a little strange to
most C/C++ programmers, but in the long run in makes the system
extremely flexible and highly extensible. Also, in terms of coding,
many of the newly DOH-based subsystems are less than half the size (in
lines of code) of the earlier C++ implementation.
2.2 Basic Types
The following built-in types are currently provided by DOH:
- String. A string of characters with automatic memory
management and high-level operations such as string replacement. In addition,
strings support file I/O operations that make it possible to use them just
about anyplace a file can be used.
- List. A list of arbitrary DOH objects (of possibly mixed types).
- Hash. A hash table that maps a set of string keys to a set of arbitrary
DOH objects. The DOH version of an associative array for all of you Perl fans.
- File. A DOH wrapper around the C FILE * structure. This is provided
since other objects sometimes want to behave like files (strings for instance).
- Void. A DOH wrapper around an arbitrary C pointer. This can be used
if you want to place arbitrary C data structures in DOH lists and hash tables.
Due to dynamic typing, all of the objects in DOH are represented by pointers
of type DOH *. Furthermore, all objects are completely
opaque--that means that the only way to access the internals of an
object is through a well-defined public API. For convenience, the following
symbolic names are sometimes used to improve readability:
- DOHString *. A String object.
- DOHList *. A list object.
- DOHHash *. A hash object.
- DOHFile *. A file object.
- DOHVoid *. A void object.
- DOHString_or_char *. A DOH String object or a raw C "char *".
It should be stressed that all of these names are merely symbolic aliases to the
type DOH * and that no compile-time type checking is performed (of course,
a runtime error may occur if you screw up).
2.3 Creating, Copying, and Destroying Objects
The following functions can be used to create new DOH objects
- NewString(DOHString_or_char *value)
Create a new string object with contents initially
set to value. value can be either a C string or a DOH string object.
- NewStringf(char *fmt, ...)
Create a new string object with contents initially set to
a formatted string. Think of this as being sprintf() combined with an object constructor.
- NewList()
Create a new list object that is initially empty.
- NewHash()
Create a new hash object that is initially empty.
- NewFile(DOHString_or_char *filename, char *mode)
Open a file and return a file object. This is a
wrapper around the C fopen() library call.
- NewFileFromFile(FILE *f)
Create a new file object given an already opened FILE * object.
- NewVoid(void *obj, void (*del)(void *))
Create a new DOH object that is a wrapper around an
arbitrary C pointer. del is an optional destructor function that will be called when the object
is destroyed.
Any object can be copied using the Copy() function. For example:
DOH *a, *b, *c, *d;
a = NewString("Hello World");
b = NewList();
c = Copy(a); /* Copy the string a */
d = Copy(b); /* Copy the list b */
Copies of lists and hash tables are shallow. That is, their contents are only copied by reference.
Objects can be deleted using the Delete() function. For example:
DOH *a = NewString("Hello World");
...
Delete(a); /* Destroy a */
All objects are referenced counted and given a reference count of 1 when initially created. The
Delete() function only destroys an object when the reference count reaches zero. When
an object is placed in a list or hash table, it's reference count is automatically increased. For example:
DOH *a, *b;
a = NewString("Hello World");
b = NewList();
Append(b,a); /* Increases refcnt of a to 2 */
Delete(a); /* Decreases refcnt of a to 1 */
Delete(b); /* Destroys b, and destroys a */
Should it ever be necessary to manually increase the reference count of an object, the DohIncref() function
can be used:
DOH *a = NewString("Hello");
DohIncref(a);
2.4 A Word About Mutability and Copying
All DOH objects are mutable regardless of their current reference
count. For example, if you create a string and then create a 1000
references to it (in lists and hash tables), changes to the string
will be reflected in all of the references. Therefore, if you need to
make any kind of local change, you should first make a copy using the
Copy() function. Caveat: when copying lists and hash tables, elements
are copied by reference.
2.5 Strings
The DOH String type is perhaps the most flexible object. First, it supports a variety of string-oriented
operations. Second, it supports many of the same operations as lists. Finally, strings provide file I/O
operations that allow them to be used interchangably with DOH file objects.
[ TODO ]
2.6 Lists
[ TODO ]
2.7 Hash tables
[ TODO ]
2.8 Files
[ TODO ]
2.9 Void objects
[ TODO ]
2.10 Utility functions
[ TODO ]
3. Types and Typemaps
Revised: Dave Beazley (8/14/00)
The representation and manipulation of types is currently in the
process of being reorganized and (hopefully) simplified. The
following list describes the current set of functions that are used to
manipulate datatypes. These functions are different than in
SWIG1.1 and may change names in the final SWIG1.3 release.
- SwigType_str(SwigType *t, char *name).
This function produces the exact string
representation of the datatype t. name is an optional parameter that
specifies a declaration name. This is used when dealing with more complicated datatypes
such as arrays and pointers to functions where the output might look something like
"int (*name)(int, double)".
- SwigType_lstr(SwigType *t, char *name).
This function produces a string
representation of a datatype that can be safely be assigned a value (i.e., can be used as the
"lvalue" of an expression). To do this, qualifiers such as "const", arrays, and references
are stripped away or converted into pointers. For example:
Original Datatype lstr()
------------------ --------
const char *a char *a
double a[20] double *a
double a[20][30] double *a
double &a double *a
The intent of the lstr() function is to produce local variables inside wrapper functions--all
of which must be reassignable types since they are the targets of conversions from a scripting
representation.
- SwigType_rcaststr(SwigType *t, char *name).
This function produces a string
that casts a type produced by the lstr() function to the type produced by the
str() function. You might view it as the inverse of lstr(). This function only produces
output when it needs to (when str() and lstr() produce different results). Furthermore, an optional
name can be supplied when the cast is to be applied to a specific name. Examples:
Original Datatype rcaststr()
------------------ ---------
char *a
const char *a (const char *) name
double a[20] (double *) name
double a[20][30] (double (*)[30]) name
double &a (double &) *name
- SwigType_lcaststr(SwigType *t, char *name).
This function produces a string
that casts a type produced by the str() function to the type produced by the
lstr() function. This function only produces
output when it needs to (when str() and lstr() produce different results). Furthermore, an optional
name can be supplied when the cast is to be applied to a specific name.
Original Datatype lcaststr()
------------------ ---------
char *a
const char *a (char *) name
double a[20] (double *) name
double a[20][30] (double *) name
double &a (double *) &name
- SwigType_manglestr(SwigType *t).
Produces a type-string that is used to identify this datatype in the target scripting language.
Usually this string looks something like "_p_p_double" although the target language
may redefine the output for its own purposes. Normally this function strips all qualifiers,
references, and arrays---producing a mangled version of the type produced by the lstr() function.
The following example illustrates the intended use of the above functions when creating wrapper
functions using shorthand pseudocode. Suppose you had a function like this:
int foo(int a, double b[20][30], const char *c, double &d);
Here's how a wrapper function would be generated using the type generation functions above:
wrapper_foo() {
lstr("int","result")
lstr("int","arg0")
lstr("double [20][30]", "arg1")
lstr("const char *", "arg2")
lstr("double &", "arg3")
...
get arguments
...
result = (lcaststr("int")) foo(rcaststr("int","arg0"),
rcaststr("double [20][30]","arg1"),
rcaststr("const char *", "arg2"),
rcaststr("double &", "arg3"))
...
}
Here's how it would look with the corresponding output filled in:
wrapper_foo() {
int result;
int arg0;
double *arg1;
char *arg2;
double *arg3;
...
get arguments
...
result = (int) foo(arg0,
(double (*)[30]) arg1,
(const char *) arg2,
(double &) *arg3);
...
}
Notes:
- For convenience, the string generation functions return a
"char *" that points to statically allocated memory living
inside the type library. Therefore, it is never necessary (and it's
an error) to free the pointer returned by the functions. Also, if you
need to save the result, you should make a copy of it. However, with
that said, it is probably worth nothing that these functions do cache
the last 8 results. Therefore, it's fairly safe to make a handful of
repeated calls without making any copies.
[TODO]
4. Parsing
[TODO]
5. Difference Between SWIG 1.1 and SWIG 1.3
[TODO]
6. Plans for SWIG 2.0
[TODO]
7. The C/C++ Wrapping Layer
Added: Dave Beazley (July 22, 2000)
When SWIG generates wrappers, it tries to provide a mostly seamless integration
with the original code. However, there are a number of problematic features
of C/C++ programs that complicate this interface.
- Passing and returning structures by value. When used, SWIG converts
all pass-by-value functions into wrappers that pass by reference. For example:
double dot_product(Vector a, Vector b);
gets turned into a wrapper like this:
double wrap_dot_product(Vector *a, Vector *b) {
return dot_product(*a,*b);
}
Functions that return by value require a memory allocation to store the result. For example:
Vector cross_product(Vector *a, Vector *b);
become
Vector *wrap_cross_product(Vector *a, Vector *b) {
Vector *result = (Vector *) malloc(sizeof(Vector));
*result = cross_product(a,b);
return result;
}
Note: If C++ is being wrapped, the default copy constructor is used
instead of malloc() to create a copy of the return result.
- C++ references. C++ references are handled exactly the same as
pass/return by value except that a memory allocation is not made for functions
that return a reference.
- Qualifiers such as "const" and "volatile". SWIG strips all
qualifiers from the interface presented to the target language.
Besides, what in the heck is "const" in Perl anyways?
- Instance Methods. Method invocations are handled as a function call in which
a pointer to the object (the "this" pointer) appears as the first argument. For example, in
the following class:
class Foo {
public:
double bar(double);
};
The "bar" method is wrapped by a function like this:
double Foo_bar(Foo *self, double arg0) {
return self->bar(arg0);
}
- Structure/class data members. Data members are handled by creating a pair
of wrapper functions that set and get the value respectively. For example:
struct Foo {
int x;
};
gets wrapped as follows:
int Foo_x_get(Foo *self) {
return self->x;
}
int Foo_x_set(Foo *self, int value) {
return (self->x = value);
}
- Constructors. Constructors for C/C++ data structures are wrapped by
a function like this:
Foo *new_Foo() {
return new Foo;
}
Note: For C, new objects are created using the calloc() function.
- Destructors. Destructors for C/C++ data structures are wrapper like this:
void delete_Foo(Foo *self) {
delete self;
}
Note: For C, objects are destroyed using free().
The creation of wrappers and various type transformations are handled by a collection of functions
found in the file Source/Swig/cwrap.c.
-
char *Swig_clocal(DataType *t, char *name, char *value)
This function creates a string containing the declaration of a local variable with
type t, name name, and default value value. This local
variable is stripped of all qualifiers and will be a pointer if the type is a reference
or user defined type.
-
DataType *Swig_clocal_type(DataType *t)
Returns a type object corresponding to the type string produced by the Swig_clocal() function.
- char *Swig_clocal_deref(DataType *t, char *name)
This function is the inverse of the clocal() function. Given a type and a name,
it produces a string containing the code needed to cast/convert the type produced by
Swig_clocal() back into it's original type.
- char *Swig_clocal_assign(DataType *t, char *name)
Given a type and name, this produces a string containing the code (and an optional cast)
needed to make an assignment from the real datatype to the local datatype produced
by Swig_clocal(). Kind of the opposite of deref().
- int Swig_cargs(Wrapper *w, ParmList *l)
Given a wrapper function object and a list of parameters, this function declares a set
of local variables for holding all of the parameter values (using Swig_clocal()). Returns
the number of parameters. In addition, this function sets the local name of each parameter
which can be retrieved using the Parm_Getlname() function.
- void Swig_cresult(Wrapper *w, DataType *t, char *resultname, char *decl)
Generates the code needed to set the result of a wrapper function and performs all of
the needed memory allocations for ANSI C (if necessary). t is the type of the
result, resultname is the name of the result variable, and decl is
a string that contains the C code which produces the result.
- void Swig_cppresult(Wrapper *w, DataType *t, char *resultname, char *decl)
Generates the code needed to set the result of a wrapper function and performs all of
the needed memory allocations for C++ (if necessary). t is the type of the
result, resultname is the name of the result variable, and decl is
a string that contains the C code which produces the result.
- Wrapper *Swig_cfunction_wrapper(char *fname, DataType *rtype, ParmList *parms, char *code)
Create a wrapper around a normal function declaration. fname is the name of the wrapper,
rtype is the return type, parms are the function parameters, and code is a
string containing the code in the function body.
- Wrapper *Swig_cmethod_wrapper(char *classname, char *methodname, DataType *rtype, DataType *parms, char *code)
- char *Swig_cfunction_call(char *name, ParmList *parms)
This function produces a string containing the code needed to call a C function.
The string that is produced contains all of the transformations needed to convert
pass-by-value into pass-by-reference as well as handle C++ references. Produces
a string like "name(arg0, arg1, ..., argn)".
Here is a short example showing how these functions could be used. Suppose you had a
C function like this:
double dot_product(Vector a, Vector b);
Here's how you might write a really simple wrapper function
ParmList *l = ... parameter list of the function ...
DataType *t = ... return type of the function ...
char *name = ... name of the function ...
Wrapper *w = NewWrapper();
Printf(w->def,"void wrap_%s() {\n", name);
/* Declare all of the local variables */
Swig_cargs(w, l);
/* Convert all of the arguments */
...
/* Make the function call and declare the result variable */
Swig_cresult(w,t,"result",Swig_cfunction(name,l));
/* Convert the result into whatever */
...
Printf(w->code,"}\n");
Wrapper_print(w,out);
The output of this would appear as follows:
void wrap_dot_product() {
Vector *arg0;
Vector *arg1;
double result;
...
result = dot_product(*arg0, *arg1);
...
}
Notice that the Swig_cargs(), Swig_cresult(), and Swig_cfunction() functions
have taken care of the type conversions for the Vector type automatically.
Notes:
- The intent of these functions is to provide consistent handling of function parameters
and return values so that language module writers don't have to worry about it too much.
- These functions may be superceded by features in the new typemap system which provide hooks
for specifying local variable declarations and argument conversions.
8. Reserved
9. Reserved
10. Guile Support
The information that used to live here has moved to the user
documentation, file Guile.html .
11. Python Support
[TODO]
12. Perl Support
[TODO]
13. Java Support
[TODO]
Copyright (C) 1999-2001
SWIG Development Team
|